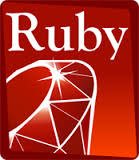
As one of my final Phase 0 challenges, I've created a cheat sheet of commonly used methods in Ruby. All of the source data for these method can originally be found from the Ruby Docs . I originally intended to list a bunch more here, and may revise this cheat sheet if I have more time next week.
Array Methods Index
#each
Calls the given block once for each element in self, passing that element as a parameter.
a = [ "a", "b", "c" ]
a.each {|x| puts x + " - test " }
=> a - test
b - test
c - test
#eachSlice
Iterates the given block for each slice of
(1..10).each_slice(3) { |a| p a }
# outputs below
[1, 2, 3]
[4, 5, 6]
[7, 8, 9]
[10]
#map
Invokes the given block once for each element of self. Creates and returns a new array containing the values returned by the block, but does not modify original array. Use map! to modify the original array.
a = [ "a", "b", "c", "d" ]
a.collect { |x| x + "!" } #=> ["a!", "b!", "c!", "d!"]
a.map.with_index{ |x, i| x * i } #=> ["", "b", "cc", "ddd"]
a #=> ["a", "b", "c", "d"]
#length
Returns the number of elements in self. May be zero.
[ 1, 2, 3, 4, 5 ].length #=> 5
[].length #=> 0